Installation and Simple Use of Redis
Install Redis on Windows
Download and install Redis: Releases · tporadowski/redis (github.com)
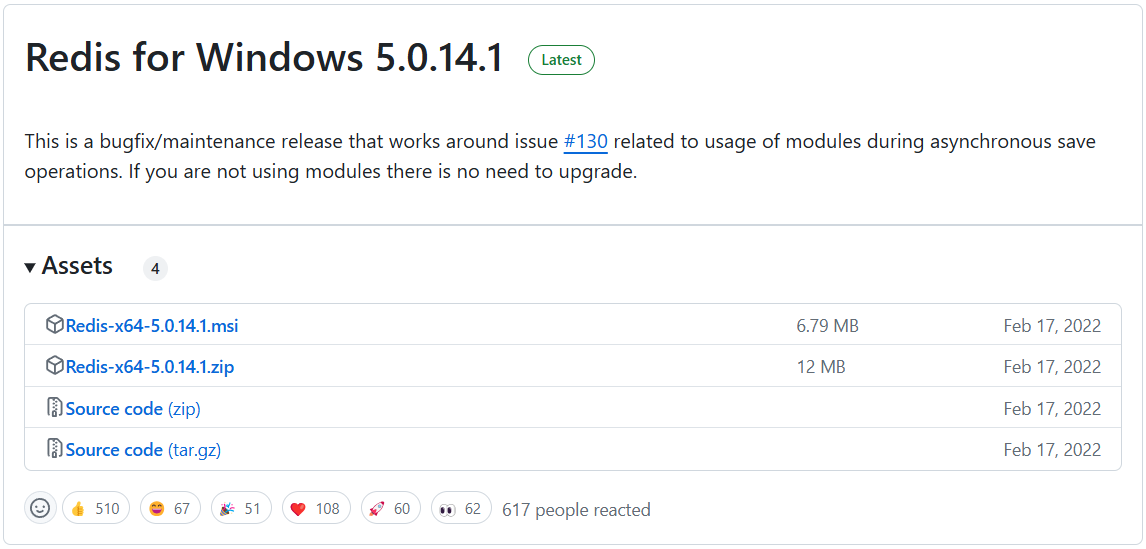
Use Redis in cmd
Open the cmd window in the Redis installation directory and execute the following command to start the service:
1 | redis-cli.exe |

Enter ping
to test whether the Redis server is running normally:
The Redis
ping
command is used by the client to send a PING to the Redis server. If the server is operating normally, a PONG will be returned.
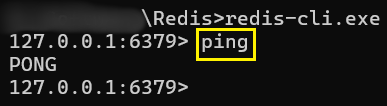
Now we can add key-value pairs to Redis:
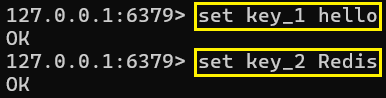
Query the key-value pairs just added:
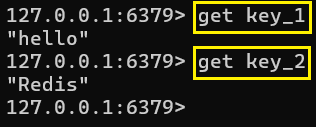
Use Redis in Python
Install Redis in Python:
1 | pip install redis |
Now we can use Redis in Python:
1 | import redis |
The output of the program is as follows:
1 | All keys in Redis before adding data: |
Use Redis in GUI Client
Redis can also be used through Redis desktop manager, here we use Redis Insight.
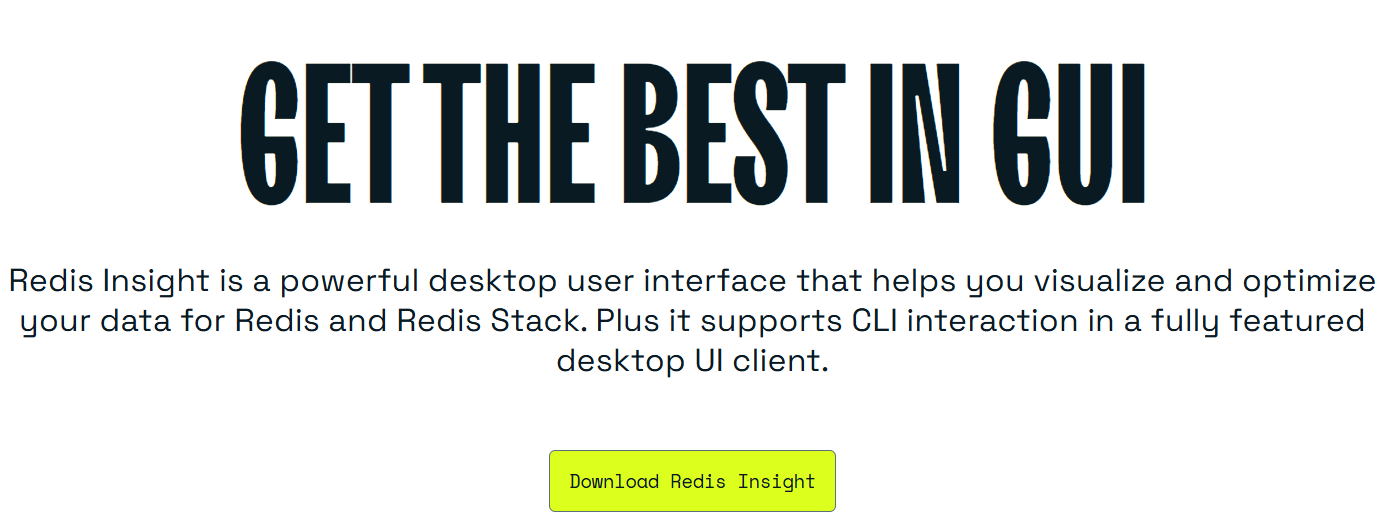
Download Redis Insight from here: https://redis.io/insight/
Now we can use Redis in the GUI:
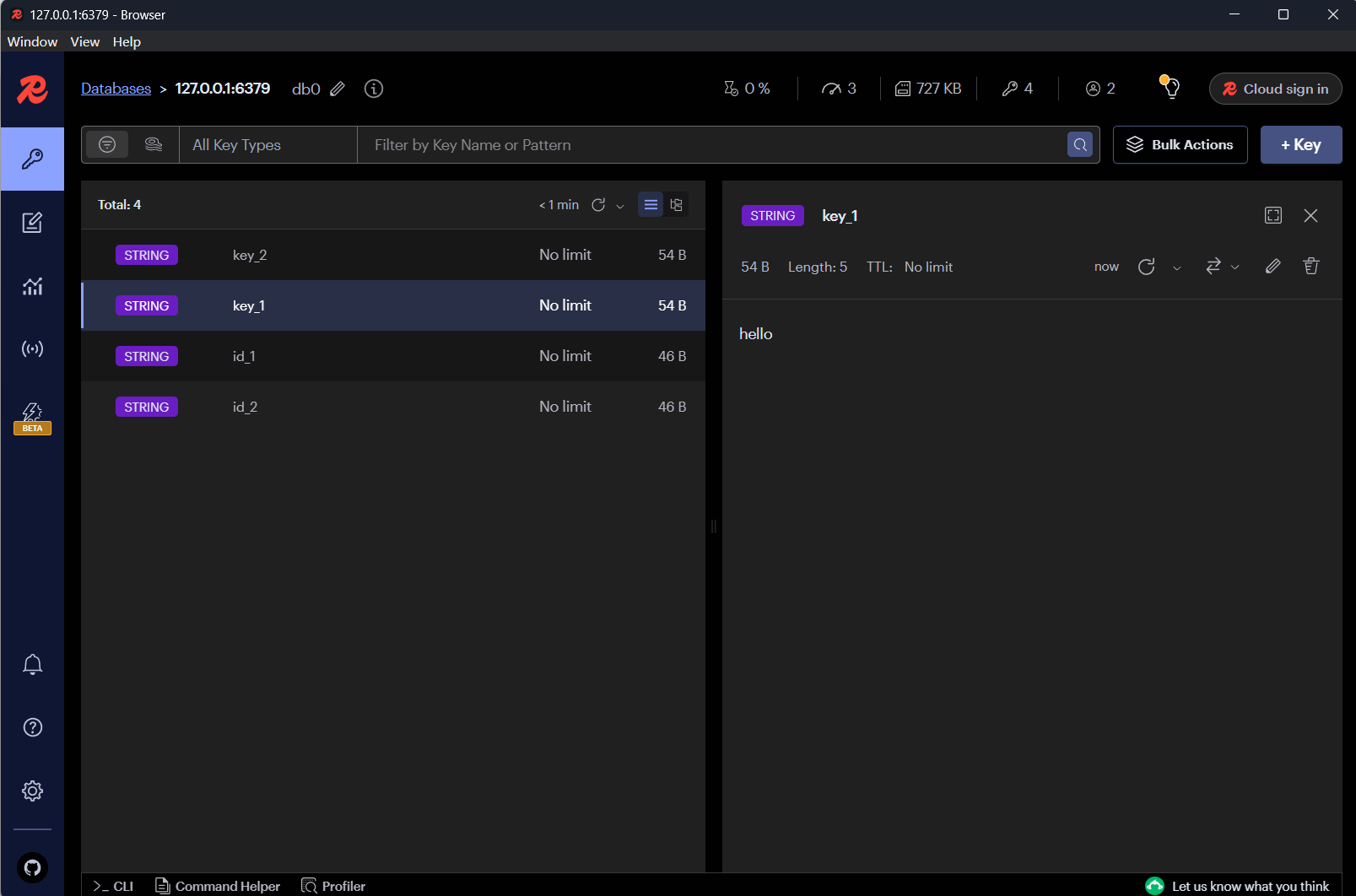