How to Convert Python Script to .exe File
Install PyInstaller
Install the Pyinstaller package via pip with the following command:
1 | pip install pyinstaller |
More information about PyInstaller: https://pyinstaller.org/en/stable/
Example
For example, we have the following script, which can generate new folders and txt files.
1 | import os |
Enter the following command in the terminal:
1 | pyinstaller -w -F generate_folders.py |
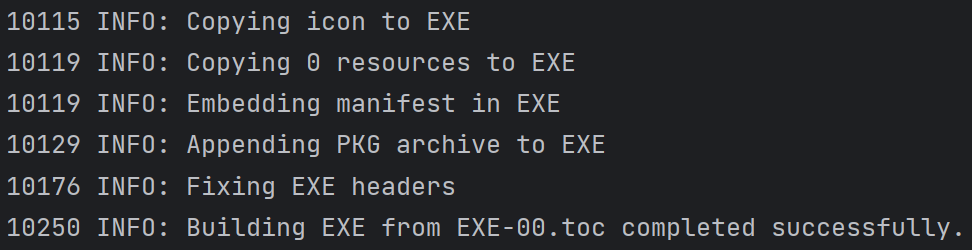
-w, --windowed, --noconsole
Windows and Mac OS X: do not provide a console window for standard i/o. On Mac OS this also triggers building a Mac OS .app bundle. On Windows this option is automatically set if the first script is a ‘.pyw’ file. This option is ignored on *NIX systems.
More info: https://pyinstaller.org/en/stable/usage.html#windows-and-mac-os-x-specific-options
-F, --onefile
Create a one-file bundled executable.
More info: https://pyinstaller.org/en/stable/usage.html#what-to-generate
Then, we can see that two new files are generated in the directory where the script is located:
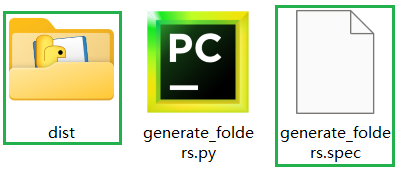
In the dist folder we can find the generated exe file:
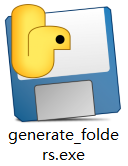
Now, double-click the generated exe file and you will find that new folders and txt files have been generated:
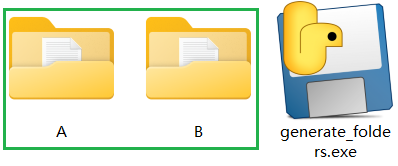
If you want to add an icon to the generated .exe file, the code is as follows:
1 | pyinstaller -F -w --icon=your_icon.ico your_code.py |
Note: Replace your_icon and your_code with your own file name.